Simple Cookie Authentication in ASP.NET Core
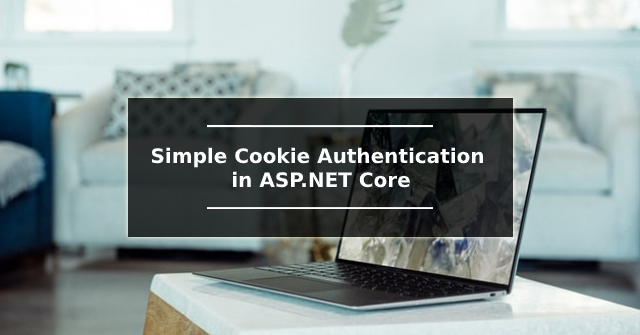
Authentication is the process of verifying that the user has access to the application. There are several ways to achieve this in ASP.NET Core. This blog is going to explain how to implement it on ASP.NET Core MVC. Gradually, we will see how to implement it Step 1: First, you need to add AddAuthentication to ConfigureServices. That registers the services required for authentication services. The following code registers the cookie authentication services. Startup.cs public void ConfigureServices( IServiceCollection services) { ... ... ... services.AddAuthentication( CookieAuthenticationDefaults .AuthenticationScheme) .AddCookie(options => { options.Cookie.Name = "SampleCookieAuth" ; options.LoginPath = "/Account/Login" ; }); services.AddControllersWithViews(); } Next, you have to add the UseAuthentication extension method above the UseAuthorization method. It adds authentication m...